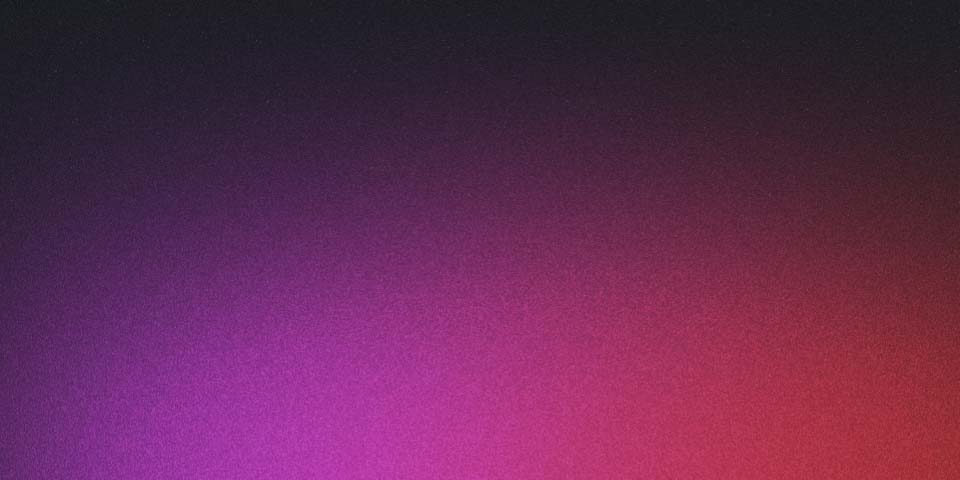
How to Add Liquibase to Existing Spring Boot Applications
Introduction
Managing database schema changes in a Spring Boot application can be challenging, especially as your project grows and the database schema evolves. For Spring Boot applications, Liquibase provides an excellent solution to manage, track, and apply database changes consistently.
In this post, we’ll guide you through integrating Liquibase into your existing Spring Boot application, enabling automatic schema management and versioning for your database.
What is Liquibase?
Liquibase is an open-source database schema change management tool. It helps you manage database changes by versioning them and applying them in a controlled, repeatable manner. Liquibase allows you to define database changes in a variety of formats, including XML, YAML, JSON, and SQL, and it can be used to:
- Generate database change logs
- Sync and track changes across environments
- Rollback database changes when necessary
- Automate schema updates during application deployment
Basic Features of Liquibase
- Database Change Logs: Liquibase uses change sets, which are individual unit changes to the database (e.g., adding a table, changing a column, inserting data).
- Version Control: Liquibase keeps track of changes and ensures that each change set is applied in order and only once.
- Multiple Formats: Liquibase supports multiple formats for defining changes (XML, YAML, SQL, JSON).
- Rollback Support: Liquibase enables you to rollback any changes or groups of changes applied to your database.
Installing Liquibase CLI
Before integrating Liquibase with your Spring Boot application, you need to install the Liquibase command-line interface (CLI). This will allow you to interact with your database and manage schema changes.
Steps to Install Liquibase CLI:
- Download Liquibase CLI:
- Visit Liquibase Downloads to get the latest version for your operating system.
- Install Liquibase:
- Follow the installation instructions based on your OS.
Once installed, you can begin using the Liquibase CLI to run essential database management commands.
Key Liquibase CLI Commands
-
generate-changelog: This command generates a changelog file based on your current database schema. It creates a changelog that you can use to version your database changes.
-
changelogSync: This command synchronizes your database with the Liquibase changelog. It updates the
DATABASECHANGELOG
table to reflect that the changesets have been applied.
Generating a Changelog
To get started with Liquibase, you need to generate a changelog from your existing database schema. This process will capture the current state of your database and create a changelog that you can use for future migrations.
Running the Generate Changelog Command
Use the following command to generate a changelog:
$ liquibase \
--url=DB_URL \
--defaultSchemaName=SCHEMA_NAME \
--changeLogFile=PATH_TO_OUTPUT_FILE.xml \
--username=DB_USERNAME \
--password=DB_PASSWORD \
generate-changelog
Example: Generating a Changelog for PostgreSQL
$ liquibase \
--url=jdbc:postgresql://localhost:5432/myapp \
--defaultSchemaName=public \
--changeLogFile=changelog/001_initial_schema.xml \
--username=postgres \
--password=secret \
generate-changelog
This will generate an XML changelog file (001_initial_schema.xml
) that contains the current structure of your database.
Syncing Changelog
Once you have generated your changelog, you’ll need to synchronize it with your database. This ensures that Liquibase knows which changes have been applied.
Running the Sync Command
$ liquibase \
--url=DB_URL \
--defaultSchemaName=SCHEMA_NAME \
--changeLogFile=PATH_TO_CHANGLOG_FILE.xml \
--username=DB_USERNAME \
--password=DB_PASSWORD \
changelogSync
Example: Syncing the Changelog
$ liquibase \
--url=jdbc:postgresql://localhost:5432/myapp \
--defaultSchemaName=public \
--changeLogFile=changelog/001_initial_schema.xml \
--username=postgres \
--password=secret \
changelogSync
This will update the DATABASECHANGELOG
table in your database, marking the changes as applied.
Organizing Liquibase Files
It’s important to keep your Liquibase files organized to maintain clarity as your project grows. Here’s a recommended structure for your Liquibase files:
db/
changelog/
db.changelog-master.yaml
db.changelog-main.yaml
db.changelog-local.yaml
schemas/
001_create_tables.sql
002_update_project_table.sql
data/
001_initial_data.sql
002_referential_data.sql
local/
001_test_data.sql
Explanation of the Folder Structure
db/changelog/
: Contains your main changelog files (YAML, XML, etc.). These files control the order of database migrations.schemas/
: Contains SQL files for schema changes, like table creation and index creation.data/
: Contains data insertion scripts.local/
: Used for data that should only be inserted in your local environment (e.g., test data).
Example: db.changelog-master.yaml
databaseChangeLog:
- include:
file: db/changelog/db.changelog-main.yaml
- include:
file: db/changelog/db.changelog-local.yaml
context: local,test
Example: db.changelog-main.yaml
databaseChangeLog:
- changeSet:
id: 1
author: cihancinar
- include:
file: schemas/001_create_tables.sql
relativeToChangelogFile: true
- changeSet:
id: 2
author: cihancinar
- include:
file: data/001_initial_data.sql
relativeToChangelogFile: true
- changeSet:
id: 3
author: cihancinar
- include:
file: schemas/002_update_project_table.sql
relativeToChangelogFile: true
Example: db.changelog-local.yaml
databaseChangeLog:
- changeSet:
id: 5
author: cihancinar
- include:
file: local/001_test_data.sql
relativeToChangelogFile: true
Running Liquibase with Spring Boot
Now that you’ve set up Liquibase and created your changelog files, you can integrate Liquibase into your Spring Boot application.
- Add Liquibase Dependency to
pom.xml
(for Maven):
<dependency>
<groupId>org.liquibase</groupId>
<artifactId>liquibase-core</artifactId>
</dependency>
- Configure Liquibase in
application.properties
:
spring.liquibase.change-log=classpath:db/changelog/db.changelog-master.yaml
spring.liquibase.enabled=true
- Run Your Spring Boot Application: On startup, Liquibase will automatically run the migrations based on the changes in your changelog files.
Conclusion
By following these steps, you’ve successfully integrated Liquibase into your Spring Boot application, enabling you to efficiently manage your database schema and migrations. Liquibase helps you keep your database in sync across environments and ensures consistent application of schema changes.
Happy coding and happy migrations!